清华大佬耗费三个月吐血整理的几百G的资源,免费分享!....>>>
摩尔斯电码(又译为摩斯密码,Morse code)是一种时通时断的信号代码,通过不同的排列顺序来表达不同的英文字母、数字和标点符号。它由美国人艾尔菲德·维尔于1837年发明。 摩尔斯电码是一种早期的数字化通信形式,但是它不同于现代只使用零和一两种状态的二进制代码,它的代码包括五种: 点、划、点和划之间的停顿、每个字符间短的停顿(在点和划之间)、每个词之间中等的停顿以及句子之间长的停顿。
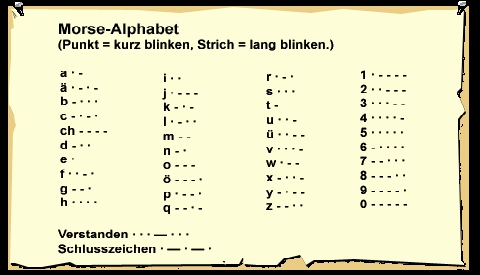
注意只可以使用英文/数字等ASCII编码127界限符中的内容并
不支持Unicode不过你可以自己修改一部分用于支持Unicode
示例代码:
public static class MorseCode // 摩尔斯电码(星际穿越) { private static volatile string[,] CodeTable = { {"A",".-"}, {"B","-..."}, {"C","-.-."}, {"D","-.."}, {"E","."}, {"E","..-.."}, {"F","..-."}, {"G","--."}, {"H","...."}, {"I",".."}, {"J",".---"}, {"K","-.-"}, {"L",".-.."}, {"M","--"}, {"N","-."}, {"O","---"}, {"P",".--."}, {"Q","--.-"}, {"R",".-."}, {"S","..."}, {"T","-"}, {"U","..-"}, {"V","...-"}, {"W",".--"}, {"X","-..-"}, {"Y","-.--"}, {"Z","--.."}, {"0","-----"}, {"1",".----"}, {"2","..---"}, {"3","...--"}, {"4","....-"}, {"5","....."}, {"6","-...."}, {"7","--..."}, {"8","---.."}, {"9","----."}, {".",".-.-.-"}, {",","--..--"}, {":","---..."}, {"?","..--.."}, {"\'",".----."}, {"-","-....-"}, {"/","-..-."}, {"(","-.--."}, {")","-.--.-"}, {"\"",".-..-."}, {"=","-...-"}, {"+",".-.-."}, {"*","-..-"}, {"@",".--.-."}, {"{UNDERSTOOD}","...-."}, {"{ERROR}","........"}, {"{INVITATION TO TRANSMIT}","-.-"}, {"{WAIT}",".-..."}, {"{END OF WORK}","...-.-"}, {"{STARTING SIGNAL}","-.-.-"}, {" ","\u2423"} }; public static string Enc(string str) { int i; string ret = string.Empty; if (str != null && (str = str.ToUpper()).Length > 0) foreach (char asc in str) if ((i = Find(asc.ToString(), 0)) > -1) ret += " " + CodeTable[i, 1]; return ret; } public static string Dec(string str) { int i; string[] splits; string ret = string.Empty; if (str != null && (splits = str.Split(' ')).Length > 0) { foreach (string split in splits) if ((i = Find(split, 1)) > -1) ret += CodeTable[i, 0]; return ret; } return "{#}"; } private static int Find(string str, int cols) { int i = 0, len = CodeTable.Length / 2; // len / rank while (i < len) { if (CodeTable[i, cols] == str) return i; i++; }; return -1; } }
使用代码:
string encry = MorseCode.Enc("China"); // 把China换成摩尔斯电码 string decry = MorseCode.Dec(encry); //把encry换成明文形式